Noun: Pure software intern coding challenge
A pure software intern coding challenge is an assessment involving the completion of predetermined programming tasks and algorithms using only text-based code. For instance, a challenge may require writing a program in Python to sort a list of numbers or reverse a string.
These challenges are widely used by tech companies to evaluate the programming abilities and problem-solving skills of intern candidates. They provide a structured way to assess candidates’ technical proficiency, identify top talent, and make informed hiring decisions.
A significant historical development in software intern coding challenges is the rise of online platforms. These platforms offer a standardized and efficient way for companies to conduct coding challenges and for candidates to demonstrate their skills.
In this article, we will explore the different types of pure software intern coding challenges, discuss their benefits, and provide tips for preparing for and excelling in these assessments.
pure software intern coding challenge
When preparing for a pure software intern coding challenge, it is essential to understand the key aspects that these challenges assess. These aspects cover various dimensions of a candidate’s technical proficiency and problem-solving abilities.
- Data structures
- Algorithms
- Object-oriented programming
- Software design
- Problem-solving
- Debugging
- Communication
- Teamwork
- Time management
- Stress management
These aspects are interconnected and essential for success in the software industry. A strong understanding of data structures and algorithms is the foundation for efficient code. Object-oriented programming principles enable the design of scalable and maintainable software. Problem-solving skills are crucial for tackling complex technical challenges. Debugging is an essential skill for identifying and fixing errors in code. Communication and teamwork are important for collaborating effectively on software projects. Time and stress management are essential for completing coding challenges under pressure.
Data structures
In the context of pure software intern coding challenges, data structures play a pivotal role in assessing candidates’ foundational understanding of computer science and their ability to design and implement efficient code. Data structures organize and store data in a systematic way, enabling efficient access and manipulation.
-
Arrays
Arrays are a fundamental data structure that stores elements of the same type in a contiguous block of memory. They provide fast random access to elements and are commonly used to represent lists, tables, and queues.
-
Linked lists
Linked lists are a linear data structure that stores data in nodes, where each node contains a value and a reference to the next node. They are useful for representing sequences of data that need to be inserted or removed frequently.
-
Stacks
Stacks are a last-in-first-out (LIFO) data structure that follows the principle of pushing and popping elements. They are used to implement recursion, function calls, and managing memory.
-
Queues
Queues are a first-in-first-out (FIFO) data structure that follows the principle of enqueueing and dequeueing elements. They are used to implement waiting lines, message queues, and job schedulers.
Understanding these fundamental data structures is essential for solving coding challenges efficiently. Candidates should be proficient in implementing these data structures in the programming language of their choice and understanding their time and space complexity characteristics.
Algorithms
In the realm of pure software intern coding challenges, algorithms play a central role in evaluating candidates’ problem-solving abilities and their understanding of computer science fundamentals. Algorithms are precise sequences of instructions that define how a task is to be accomplished, and they are the cornerstone of efficient and effective software development.
Coding challenges often involve solving algorithmic problems, such as searching for an element in an array, sorting a list of numbers, or finding the shortest path in a graph. These challenges test candidates’ ability to devise logical solutions, analyze their time and space complexity, and implement them efficiently in the programming language of choice.
Real-life examples of algorithms in pure software intern coding challenges include implementing a merge sort algorithm to sort a list of integers, using a binary search algorithm to find a specific element in an array, or designing a dynamic programming algorithm to solve a knapsack problem. Understanding these algorithms and their underlying principles is crucial for success in coding challenges and, more importantly, in the development of high-quality software.
The practical applications of understanding algorithms extend far beyond coding challenges. Algorithms are used in a wide range of software applications, from search engines and social media platforms to operating systems and scientific simulations. By mastering algorithms, software engineers gain the ability to design and implement efficient solutions to complex problems, leading to improved software performance, reduced development time, and enhanced user experience.
Object-oriented programming
Within the realm of pure software intern coding challenges, object-oriented programming (OOP) stands as a critical aspect, testing candidates’ proficiency in designing and implementing modular, maintainable, and extensible software solutions.
-
Encapsulation
Encapsulation is the bundling of data and methods into a single unit, known as an object. This allows for data hiding, where the internal state of an object is protected from external access, enhancing security and reducing the risk of unintended modifications.
-
Inheritance
Inheritance enables the creation of new classes (derived or child classes) from existing classes (base or parent classes). Derived classes inherit the properties and behaviors of their parent classes, promoting code reusability, reducing redundancy, and facilitating the extension of existing functionality.
-
Polymorphism
Polymorphism allows objects of different classes to respond to the same message in a uniform manner. This is achieved through method overriding, where derived classes can provide their own implementation of methods inherited from parent classes, enabling flexible and extensible code.
-
Abstraction
Abstraction involves creating classes and interfaces that define a clear and concise contract without exposing implementation details. This promotes loose coupling between components, making it easier to maintain and modify the codebase while preserving its overall functionality.
These facets of OOP are frequently tested in pure software intern coding challenges. Candidates are expected to demonstrate their understanding of OOP concepts by designing classes with appropriate data structures and methods, utilizing inheritance and polymorphism to achieve code reusability and flexibility, and applying abstraction to create maintainable and extensible software systems.
Software design
In the context of pure software intern coding challenges, software design occupies a crucial position, serving as the cornerstone upon which successful solutions are built. It involves the application of engineering principles to create software that is not only functionally correct but also maintainable, extensible, and scalable.
Software design encompasses various aspects, including architecture, data structures, algorithms, and coding standards. A well-designed software system exhibits modularity, allowing for independent development and maintenance of its components. It incorporates appropriate data structures to efficiently store and retrieve data, and employs suitable algorithms to perform computations and solve problems. Additionally, it adheres to established coding standards to ensure consistency, readability, and adherence to best practices.
Real-life examples of software design in pure software intern coding challenges include designing a class hierarchy to represent a system’s entities, defining interfaces to facilitate communication between components, and implementing design patterns to solve common software engineering problems. Understanding software design principles enables candidates to create software solutions that are not only correct but also well-structured, flexible, and easy to maintain.
The practical applications of software design extend beyond coding challenges and permeate the entire software development lifecycle. By adopting sound software design principles, engineers can create software systems that are easier to modify, extend, and maintain, leading to reduced development costs, faster time-to-market, and improved software.
Problem-solving
Within the realm of pure software intern coding challenges, problem-solving stands as the cornerstone, demanding candidates to demonstrate their ability to analyze, understand, and devise solutions to complex computational problems. Coding challenges often present intricate scenarios that require candidates to apply their problem-solving skills to develop efficient and effective algorithms and data structures.
Problem-solving is a critical component of pure software intern coding challenges as it evaluates a candidate’s fundamental understanding of computer science concepts and their ability to apply them in practice. It goes beyond mere coding proficiency and delves into the candidate’s analytical thinking, logical reasoning, and ability to break down problems into manageable components.
Real-life examples of problem-solving in pure software intern coding challenges include designing an algorithm to find the shortest path in a graph, developing a data structure to store and efficiently retrieve a large dataset, or implementing a sorting algorithm that optimizes for both time and space complexity. These challenges test candidates’ ability to think critically, identify patterns, and apply appropriate techniques to solve problems.
The practical applications of understanding problem-solving extend far beyond coding challenges and permeate the entire software development lifecycle. Software engineers are constantly faced with complex problems that require innovative solutions. By honing their problem-solving skills, they gain the ability to approach challenges systematically, analyze requirements, and develop elegant and efficient solutions that meet the needs of users and businesses.
Debugging
In the realm of pure software intern coding challenges, debugging plays a pivotal role in assessing candidates’ ability to identify and resolve errors in their code. As candidates strive to complete coding challenges under time constraints, errors are inevitable. Debugging effectively demonstrates their problem-solving skills, attention to detail, and ability to think logically.
Debugging is a critical component of pure software intern coding challenges, as it evaluates candidates’ ability to analyze error messages, trace the execution of their code, and identify the root cause of any issues. It requires a systematic approach, where candidates need to understand the expected behavior of their code, compare it with the actual behavior, and pinpoint the source of discrepancies.
Real-life examples of debugging within pure software intern coding challenges include identifying and fixing errors in data structures, resolving issues with algorithm implementation, and handling exceptions gracefully. Candidates are expected to demonstrate proficiency in using debugging tools, such as print statements, logging, and debuggers, to locate and fix errors efficiently.
The practical applications of understanding debugging extend beyond coding challenges and are essential for software engineers in industry. Software systems are complex, and errors can arise from various sources. By mastering debugging techniques, engineers can systematically identify and resolve issues, ensuring the reliability, stability, and performance of software products.
Communication
In the world of pure software intern coding challenges, communication plays a subtle yet critical role in assessing candidates’ abilities. Beyond technical prowess, effective communication skills are essential for conveying solutions clearly and concisely, fostering collaboration, and ultimately succeeding in the challenge.
Firstly, communication is vital for conveying solutions accurately. Coding challenges often require candidates to explain their approach and thought process, both in written and verbal form. Clear and well-organized communication ensures that the evaluator can follow the candidate’sand understand the rationale behind their solution.
Secondly, communication is crucial for fostering collaboration. Many coding challenges involve o programming or group discussions, where candidates must effectively communicate their ideas, resolve conflicts, and coordinate their efforts. Strong communication skills enable candidates to work harmoniously, share knowledge, and contribute to a shared understanding of the problem and its solution.
Real-life examples of communication within pure software intern coding challenges include explaining the design choices behind a particular algorithm, discussing the pros and cons of different approaches with fellow candidates or the evaluator, and seeking clarification on problem requirements or constraints.
The practical applications of understanding the connection between communication and pure software intern coding challenges extend beyond the immediate challenge itself. In the software industry, effective communication is paramount for successful teamwork, knowledge sharing, and delivering high-quality software products. By honing their communication skills, software engineers can navigate complex technical discussions, contribute to design decisions, and collaborate effectively with colleagues and clients.
Teamwork
In the context of pure software intern coding challenges, teamwork plays a significant role in evaluating candidates’ ability to collaborate effectively and contribute to a shared solution. Teamwork challenges assess candidates’ communication, problem-solving, and interpersonal skills, which are essential for success in a collaborative software development environment.
-
Communication
Effective communication is crucial for successful teamwork in coding challenges. Candidates must be able to clearly convey their ideas, both verbally and in writing, to ensure that all team members are on the same page. They should also be able to actively listen to others and provide constructive feedback.
-
Problem-solving
Teamwork challenges often require candidates to solve complex problems that cannot be easily solved by a single individual. Candidates must be able to work together to identify the problem, brainstorm solutions, and implement the best solution.
-
Interpersonal skills
Interpersonal skills are essential for creating a positive and productive team environment. Candidates must be able to work harmoniously with others, even under pressure. They should also be able to resolve conflicts and build consensus.
-
Time management
Time management is critical in teamwork challenges, as candidates must be able to effectively allocate their time and resources to complete the challenge within the given timeframe. They should be able to prioritize tasks and delegate responsibilities to ensure that the team meets its goals.
Overall, teamwork challenges in pure software intern coding challenges provide a valuable opportunity for candidates to demonstrate their ability to work effectively in a collaborative environment. These challenges assess candidates’ communication, problem-solving, interpersonal, and time management skills, which are essential for success in the software industry.
Time management
In the context of pure software intern coding challenges, time management plays a pivotal role in a candidate’s success. Coding challenges often impose strict time constraints, necessitating efficient utilization of the allotted time to maximize performance. Effective time management encompasses various aspects, including:
-
Task prioritization
Candidates must prioritize tasks based on their importance and urgency, ensuring that critical tasks are completed within the given timeframe. Real-life examples include identifying the most critical algorithms to implement or prioritizing bug fixes based on their impact on the overall solution.
-
Time allocation
Candidates should allocate time wisely to different tasks, considering the complexity and time requirements of each. Real-life examples include allocating more time to design complex algorithms or budgeting time for testing and debugging.
-
Progress tracking
Regularly monitoring progress allows candidates to identify potential bottlenecks and adjust their time allocation accordingly. Real-life examples include tracking the completion of coding tasks or the time taken to resolve errors.
-
Stress management
Coding challenges can be stressful, and effective time management helps candidates manage stress by avoiding last-minute rushes and maintaining a sense of control over their work. Real-life examples include taking short breaks to clear their mind or practicing relaxation techniques.
Overall, strong time management skills enable candidates to complete coding challenges within the given timeframe, demonstrate their ability to work under pressure, and produce high-quality solutions. By mastering time management techniques, candidates can improve their performance in coding challenges and enhance their overall problem-solving abilities.
Stress management
In the context of pure software intern coding challenges, stress management plays a crucial role in maintaining focus, enhancing performance, and achieving optimal results. Coding challenges often induce stress due to time constraints, problem complexity, and the pressure to perform well. Effective stress management techniques help candidates overcome these challenges and perform to the best of their abilities.
Stress management is a critical component of pure software intern coding challenges because it enables candidates to manage their emotions, maintain composure, and make rational decisions under pressure. Real-life examples of stress management within coding challenges include practicing relaxation techniques such as deep breathing or meditation to calm nerves and reduce anxiety. Additionally, candidates can engage in physical activities or hobbies outside of the challenge to relieve stress and maintain a healthy work-life balance.
The practical applications of understanding the connection between stress management and pure software intern coding challenges extend beyond the immediate challenge itself. In the software industry, engineers often face stressful situations, including tight deadlines, complex problem-solving, and the pressure to deliver high-quality products. By mastering stress management techniques, software engineers can effectively cope with these challenges, maintain their productivity, and produce better results.
In summary, stress management is an essential aspect of pure software intern coding challenges, as it allows candidates to perform optimally under pressure and make informed decisions. By understanding the importance of stress management and practicing effective techniques, candidates can overcome anxiety, improve their problem-solving abilities, and enhance their overall performance in coding challenges and beyond.
Frequently Asked Questions (FAQs)
This section provides answers to commonly asked questions and clarifies essential aspects of pure software intern coding challenges.
Question 1: What is the purpose of a pure software intern coding challenge?
Answer: A pure software intern coding challenge assesses a candidate’s programming skills and problem-solving abilities in a structured and standardized environment. It helps recruiters evaluate candidates’ proficiency in data structures, algorithms, software design, and other core computer science concepts.
Question 2: What types of tasks are typically included in a pure software intern coding challenge?
Answer: Challenges may involve implementing algorithms, designing data structures, solving logic puzzles, or writing code to meet specific requirements. Candidates are often given a set of test cases to verify the correctness of their solutions.
Question 3: How are pure software intern coding challenges evaluated?
Answer: Solutions are typically evaluated based on factors such as code efficiency, correctness, adherence to coding standards, and the overall approach taken by the candidate.
Question 4: What is the importance of time management in pure software intern coding challenges?
Answer: Time management is crucial as challenges often have strict time limits. Candidates must prioritize tasks, allocate time effectively, and manage stress to complete the challenge within the given timeframe.
Question 5: How can I prepare for a pure software intern coding challenge?
Answer: Practice coding regularly, focus on mastering data structures and algorithms, familiarize yourself with the programming language and tools, and manage your time effectively during the challenge.
Question 6: What are the benefits of participating in pure software intern coding challenges?
Answer: Challenges provide an opportunity to showcase your skills, gain feedback on your solutions, and enhance your problem-solving abilities. They can also help you identify areas for improvement and prepare you for the demands of a software engineering role.
These FAQs provide insights into the nature, purpose, and preparation for pure software intern coding challenges. As you delve deeper into this topic, you will gain a comprehensive understanding of the challenges involved and the strategies for success.
The next section will explore additional tips and techniques to excel in pure software intern coding challenges.
Tips for Excelling in Pure Software Intern Coding Challenges
This section provides practical tips to enhance your performance and maximize your chances of success in pure software intern coding challenges.
Tip 1: Practice Regularly: Consistent practice is essential to improve your programming skills and sharpen your problem-solving abilities.
Tip 2: Focus on Data Structures and Algorithms: Develop a strong foundation in data structures and algorithms, as they form the core of many coding challenges.
Tip 3: Master a Programming Language: Choose a programming language and become proficient in its syntax, libraries, and tools.
Tip 4: Understand the Problem Statement: Carefully analyze the problem statement to fully grasp the requirements and constraints.
Tip 5: Design an Efficient Solution: Plan your solution before writing any code, considering time and space complexity.
Tip 6: Test Your Code Thoroughly: Write comprehensive test cases to verify the correctness and robustness of your code.
Tip 7: Manage Your Time Effectively: Allocate your time wisely to complete the challenge within the given timeframe.
Tip 8: Seek Feedback and Learn: Review your solutions, analyze feedback, and identify areas for improvement.
By following these tips, you can significantly enhance your performance in pure software intern coding challenges. They will help you demonstrate your technical proficiency, problem-solving abilities, and attention to detail, increasing your chances of success and showcasing your readiness for a software engineering role.
The final section of this article will provide insights into the broader context of pure software intern coding challenges, exploring their significance in the software industry and their role in identifying and nurturing talented software engineers.
Conclusion
In exploring the multifaceted nature of pure software intern coding challenges, this article has illuminated their significance as a standardized assessment tool for evaluating candidates’ technical proficiency and problem-solving skills. These challenges provide a structured environment for showcasing expertise in data structures, algorithms, software design, and other core computer science concepts.
Key insights gleaned from this exploration include the importance of time management, the need for thorough preparation in data structures and algorithms, and the value of seeking feedback to facilitate continuous growth. Understanding these aspects enables candidates to approach coding challenges strategically, maximizing their chances of success and demonstrating their readiness for the demands of a software engineering role.
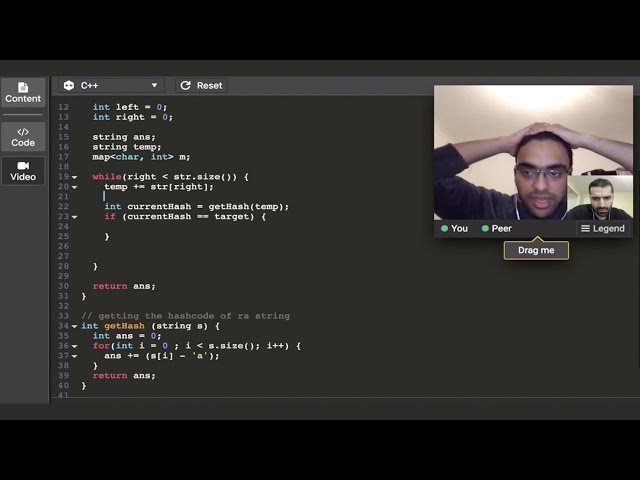